My good friend and prolific iOS app developer _David Smith has released another new app. This one, Activity++, shows you your activity history that your Apple Watch has gathered. Sure, the Activity app does the same, but Activity++ is a vast improvement.
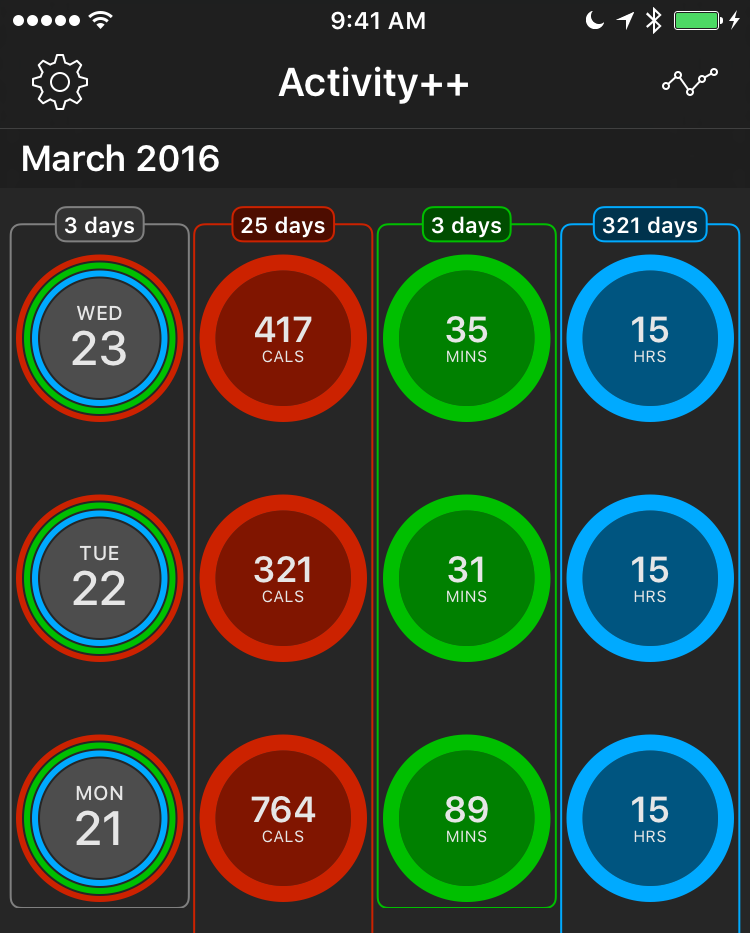
The app is a long list of your days, from most recent to least recent, stacked vertically. What I really love about the app are the statistics it shows. In the main screen, you can see how long you’ve maintained a run of one of your circles. In the example above, the last three days running I’ve hit all three goals; the last 25 I’ve hit my move goal, the last 3 I’ve hit my exercise goal, and the last 321 I’ve hit my stand goal.
I am the Blue Ring Stud, after all.
Tapping on any of these circles causes this awesome animation to happen:
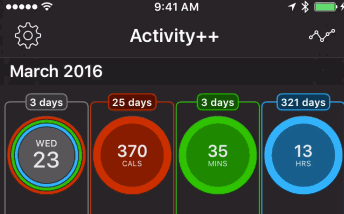
I could watch it all day.
Furthermore, there’s a statistics screen that shows you even more about how you’re doing:
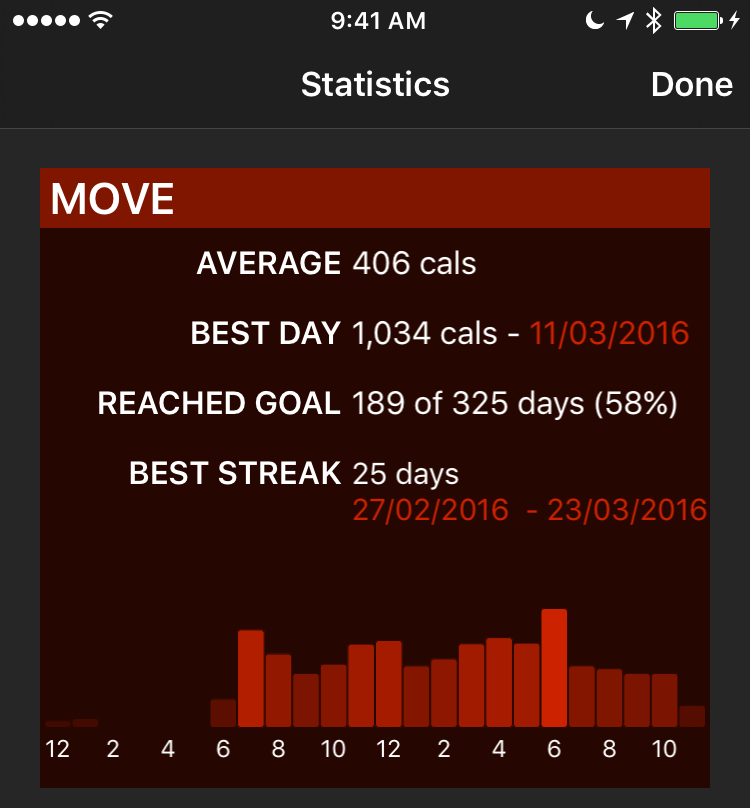
Not stopping there, David added one other great feature: “Rest Days”. If you’ve reached your goal for six consecutive days, one missed/rest day will not stop your streak.
Before you claim my Blue Ring Stud status is a farce, I will note that even without rest days, I had a 251-day streak. Damn you, flights back from the west coast.
As a final note, Activity++ also includes a handy Watch complication that makes it super easy to see the current state of your three goals, as a bar graph.
Activity++ makes your health data easy to digest, and your statistics quick to find. I’ve found this is already encouraging me to do anything I can to keep my streaks alive.
David is a dear friend, but I’d recommend Activity++ highly even if he wasn’t.
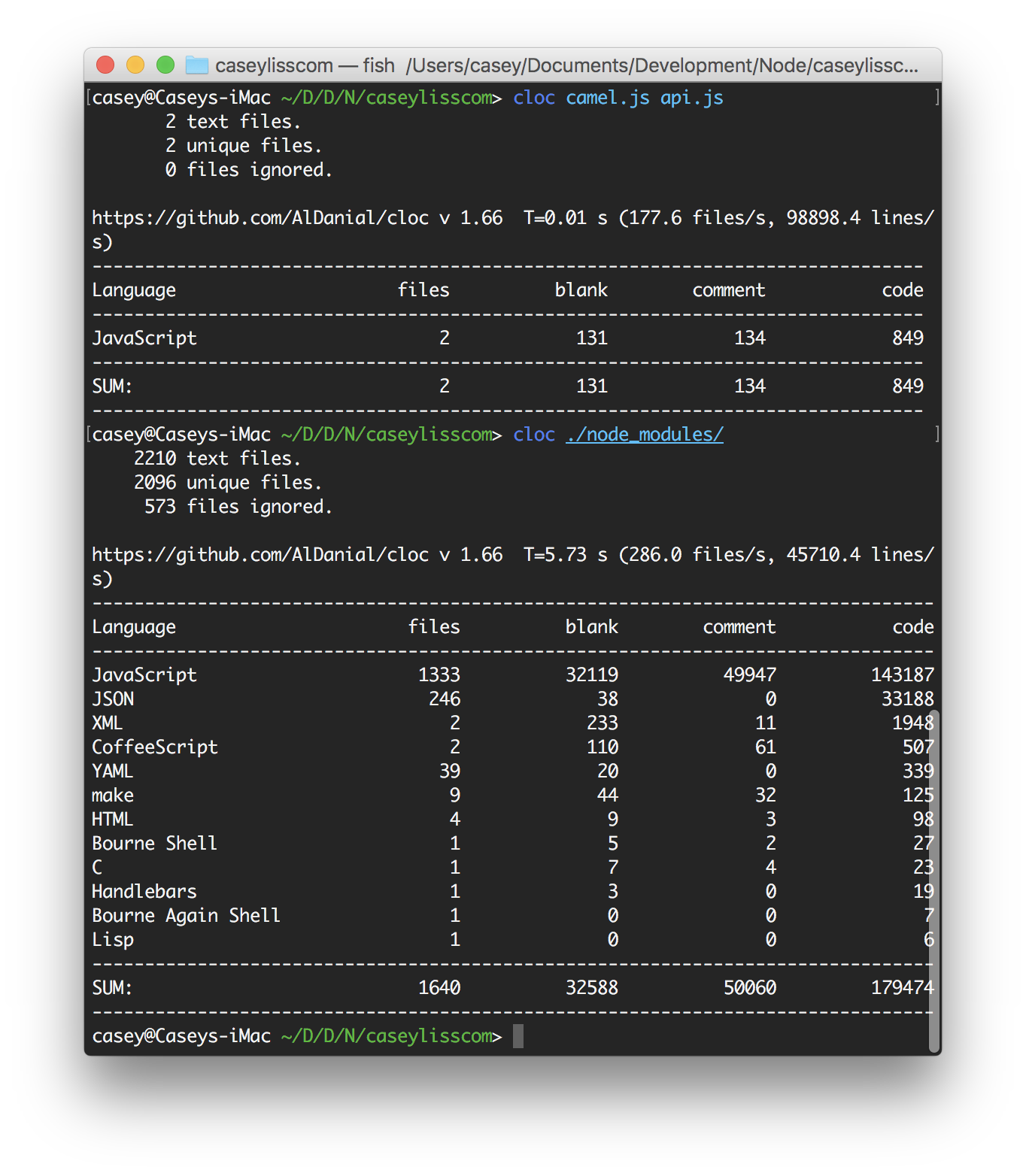
Camel itself is around 900 lines of code.
It takes nearly 200,000 lines of dependencies.
I like Node.js quite a lot, and I plan to stick with it for this blog for the foreseeable future. However, I can’t help but question my own choices.
Today I joined Christina Warren, James Thomson, and Dan Moren on this week’s Clockwise. As always, four of us, four tech topics, less than 30 minutes.
This week’s episode included the appeal (or not) of Apple News, how social media and forensics could have dramatically changed high-profile court cases, ways to intertwine the real and virtual worlds, and whether or not we would appear on the show Techno-Hoarders.
I always love to do Clockwise, as it’s so different from every other show I’ve been on. Do give it a listen.
It is an impossible task to describe what being a parent is like. That doesn’t stop me from trying though.
Yesterday, on a flight back home from a trip to Las Vegas, it occurred to me how different my life is now that I’m a father.
Once you become a parent, every time your plane jostles, you freak out. You suck your breath in. You hold on tighter, as if that will somehow keep you safer, in your flying tube, 35,000 feet up.
Every time there’s a sudden shift, you take stock. The extra life insurance you wanted to look into but completely forgot about? You remember it now. Your will that you know you should write but haven’t yet? You kick yourself for it.
It’s not that things didn’t matter to me before. It’s not that I don’t care about Erin. I have the utmost confidence Erin would be fine. In fact, I also know that she’d be fine raising Declan by herself.
But she shouldn’t have to. That’s what keeps me up at night.
That’s what makes me uncomfortable flying. The uncertainty, the lack of control, and the fear. The fear that I’ll leave them alone, bewildered, and with a pile of new problems. A mountain of messes to clean up that they didn’t make, and they don’t deserve.
That’s what being a parent is like. At least, that’s what it’s like on a turbulent flight across the country, in an eery grey fog, with everything you care about in the world on the planet thousands of feet below you.
Oklahoma high school teacher Steven Wedel writes an absolutely scathing open letter to local lawmakers and voters. Much of it rings true in other states. As the husband of a teacher, I know this all too well.
…[politicians] keep passing mandates to make us better while taking away all the resources we need just to maintain the status quo. We fear that our second jobs will prevent us from grading the papers or creating the lesson plans we already have to do from home. We fear our families will leave us because we don’t have time for them.
It tears at my heart to know that my best teacher cries over the dilemma she’s facing right now. She loves her job, but she’s afraid she can no longer afford to continue in this profession. It’s something we’re all dealing with. How far will you push us?
Not only do we not pay teachers an appropriate salary, we steadfastly refuse to fund our schools appropriately.
In my new gig, my first assignment has been to work on a proof-of-concept app that demonstrates a new architecture we’re considering. (If you care, a combination of VIPER and RxSwift.)
This new app is written entirely in Swift. I’m new to Swift.
Taking inspiration from Brent Simmons’s Swift Diary, there are a few things about Swift that are deserving of praise and I plan to call out as I’m learning the language.
Today’s case study: Enumerations.
Enumerations are not a new construct; they’ve been in most languages I’ve worked with. Generally speaking, they look something like this, in most C-derived languages:
enum LibraryBookState {
CheckedIn,
CheckedOut
}
Think of it as saying “For a library book, it can either be CheckedIn
or
CheckedOut
. Nothing else.”
As with life, things are rarely that simple.
In this contrived example, it’s useful to know that the library book is checked
out to someone, but to whom? We’d need another variable to track the patron
the book is checked out to. So, if we were designing a Book
class in Swift,
it may look like this:
class Book {
var status: LibraryBookState
var checkedOutTo: Patron?
var title: String
var author: String
}
That’s a little, well, weird, if you think about it. The patron the book is
checked out to is really part of the state of the book. A part of the state
that we’re capturing in the LibraryBookState
enumeration.
Wouldn’t it be nice if we could keep track of who the book is checked out to
within the LibraryBookState
enumeration?
In Swift, we can. All thanks to associated values.
We can write a Swift version of our enumeration like so:
enum LibraryBookState {
case CheckedIn
case CheckedOut(patron: Patron)
}
Note the change in CheckedOut
: now it looks like a function call. Though
syntactically a little curious, CheckedOut
is still just an enumeration
value. However, it has a super power: it carries with it an associated
value.
The only way to set a variable to the CheckedOut
enumeration value is
to also provide the associated Patron
along with it.
let casey = Patron(name: "Casey Liss")
var state = .CheckedOut(patron: casey)
Similarly, when we are using a switch
to evaluate the value of that
enumeration, we can get the value back out:
switch state {
case .CheckedIn:
// ...
case .CheckedOut(let p):
// p is the associated Patron
}
Associated values are a perfect solution to a problem I didn’t know I had.
To return to our Book
class, we can now simplify it:
class Book {
var status: LibraryBookState
var title: String
var author: String
}
We no longer need checkedOutTo
since we’re capturing that within
LibraryBookState
. The revised class is much cleaner, and more
directly represents the way we wish to model a book.
I’ve found associated values are used very frequently for the results of network requests. Many take a form like this:
enum FetchBookResult {
case .Success(book: Book)
case .Failure(error: ErrorType)
}
Using enumerations in this way makes it so much easier to return a value from a function, as you don’t have to do any awkward dancing with tuples or multiple callbacks or equivalent:
func fetchBook(id: Int) -> FetchBookResult {
// ...
}
Enumerations in Swift have many other tricks as well:
- Properties
- Methods
- Extensions
- Protocols (think
interface
s)
It’s stunning how powerful support for these paradigms makes enumerations.
I’m only three weeks in, but so far Swift is really impressing me. It’s bringing a joy to development that I haven’t felt in quite some time. I often feel like I’m fighting my own ignorance; I rarely feel as though I’m fighting the language.
There’s a lot in flux with Swift these days, which makes many people I deeply respect shy away from it. That may come to burn us, if we try to go all-in on Swift; especially once Swift 3 comes along. However, I’m very hopeful and excited for the future, as it’s clear Swift is a language that is somehow able to be both well-considered and improving rapidly.
If memory serves, I met Dave Wiskus at Çingleton in 2013. I had heard the name numerous times, as I was starting to really travel in the same circles. I knew him as a designer, and frequent speaker. We hung out a bit, and I like to think, clicked pretty quickly.
Through a couple WWDCs after, and at CocoaConf DC 2014, we got to know each other better and better. For a time, we worked together. I got to learn that, as with almost anyone you meet, there’s way more to Dave Wiskus than meets the eye. We became as close as new friends, separated by a few hundred miles, can reasonably be expected to be.
I knew that Dave had dabbled with music on and off throughout his life. I also knew his current career was as a designer, speaker, and producer. Over the last year or so, I noticed him getting more and more serious about his side project, Airplane Mode.
Late last year, Dave explained how serious he really was.
Today, Airplane Mode released their first studio EP, Amsterdam. A commendable achievement, but one that is not altogether remarkable.
Dave being Dave, and Airplane Mode being Airplane Mode, they did more.
In addition to being available on iTunes and Spotify, the album is also available in book form. (Apple links are affiliate links)
It’s a quick read—it took me well under half an hour—but it tells the story of how Amsterdam came to be. The story of what motivated this quartet of musicians to perform this quartet of songs that is now an EP.
The book goes further; Dave explains:
Over time, as we make music videos or play shows to support the album, we can update the iBook with new content. In the digital age there’s no reason why a record can’t be a living document.
Like so many truly clever ideas, this one seems so obvious, but only in retrospect. Though the only thing I can play with any efficacy is the stereo, I still wish I had thought of it.
What’s more, it’s only $4.
Go check it out. Between the album and the book it’s an hour of your life. I can think of many worse ways to spend $4 and 60 minutes.
Today, I start a new job.
I was at my prior employer for over three and a half years. My time there was, on the whole, wonderful. I’ve learned a ton—both what to do and what not to do—and am thankful for the experience. Should you need some .NET development work done, including but not limited to work with Sitecore, I’ll send you their way.
During my tenure, working as a .NET developer, I was always trying to find
a way to, well,
pivot
my career into iOS development. I was lucky enough to
do one iOS project while at the old gig, but that was it. The rest of the time,
I was working in C# as usual. I like C# quite a lot, but my heart isn’t really
in it anymore.
Today, I join a local firm as an iOS developer. A dream of mine since I went to my first WWDC in 2011 is finally realized. Five years on, I’m finally walking the walk I’ve been talking about.
Just as exciting, the company I’m working for is not consulting. I’ve been working in consulting and/or government contracting (in many ways the same beast) since 2006. For a decade, I’ve been at the whims of various clients. Some of those clients—such as my last, actually—are wonderful. Some of these clients… are not.
The advantage of consulting is, if you don’t like what you’re working on (or who you’re working with), just wait a few months, and it’ll go away.
The disadvantage of consulting is, if you like what you’re working on (or who you’re working with), just wait a few months, and it’ll be taken from you.
I’m excited to see what the grass on the other side of the fence is like.
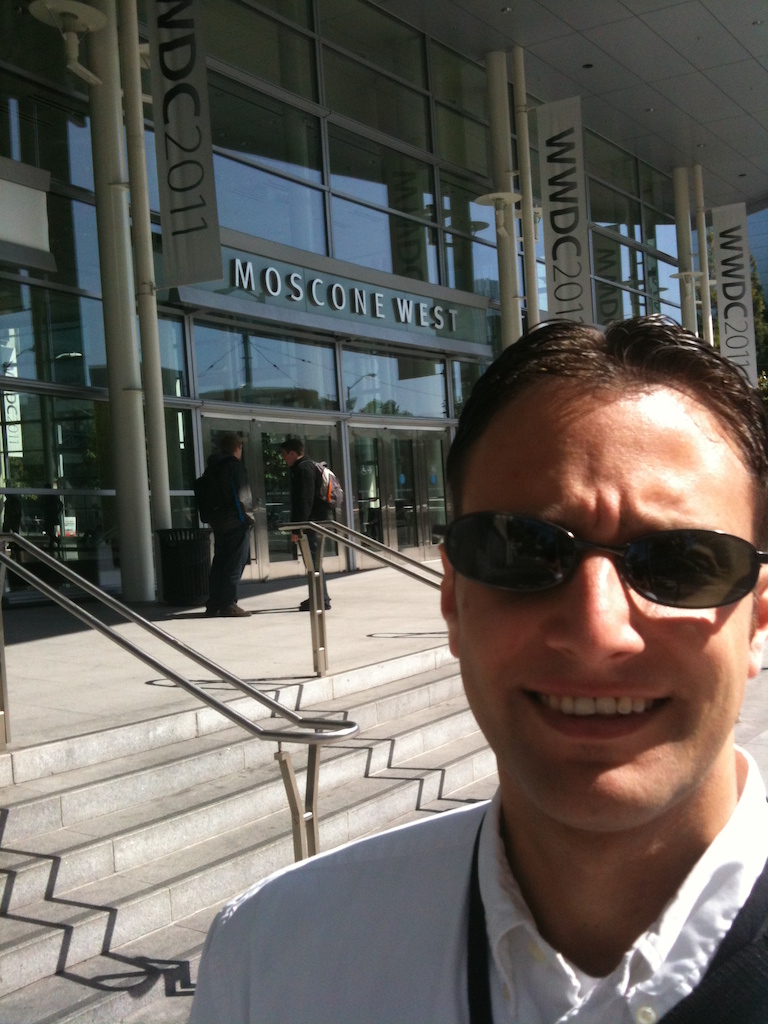
I’m absolutely petrified. I’m not a professional iOS developer… until today. Though I’ve been dabbling in it for years now, this is out of my comfort zone. I’ve spent the last few days looking at the Swift book, and I’ve been listening to Under the Radar, Mobile Couch, and Core Intuition, among others. I’m immersing myself as much as I can, but there’s no way to learn like trial by fire.
I’m so excited for this opportunity, made possible in no small part by some great friends. I’m glad to have some familiar faces to welcome me at this new gig. I’m nervous as hell that I won’t pick it up as quickly as I want to.
More than anything, I’m so stoked to be here. It’s been a long time coming.
As I write this, I have yet to speak about this job change on either of my podcasts. I know that Myke and I will be discussing this at length on the forthcoming episode of Analog(ue). When that episode is published, it will be available here. (Until then, around the 21st, that link will be broken.)
It’s time to throw caution to the wind. It’s time to be brave. It’s time to step away from the safety net.
Let’s do this.
On today’s episode of Clockwise, I joined Dan Moren, Aleen Simms, and Jason Snell to discuss four tech topics, all within 30 minutes.
This week’s episode includes streaming services we rely on, home automation we’re proud of, rumors of Apple moving toward exclusive video, and great customer service stories.
As I’ve said in the past, Clockwise is one of my favorite shows to guest on. It always keeps me on my toes, and I’m always stunned how much we can get through in so little time.
Tonight, after dinner, I was sitting near Declan trying to catch up with the Apple earnings call that was in progress. He was a few feet away, content and entertaining himself. A few minutes later, I hear him laughing hysterically. Erin is across the room, hiding, pretending she is going to sneak up on him.
I immediately join in the fun, pretending that I’ll protect Declan from Erin’s attacks. He clings to me, laughing and wailing in the happy way only an infant or toddler can.
Declan turns his head back, looking to see if Erin has moved closer while he was clinging to me. Sometimes she has, sometimes not. No matter what, once Declan locates her again, it’s back to the screams of both surprise and delight. He turns away from her, buries his head in my shoulder, clinging for dear life against the would-be attacker.
Erin is laughing. I’m giggling. Declan is nearly at the point of needing to take a break so that he can breathe he’s having so much fun.
Suddenly, like a freight train, I realize, this is that moment.
When we were having troubles conceiving, I always dreamed about what it would be like to play with my son or daughter. To giggle with them, to chase them, to be chased by them. To hear my wife and son chortling as they take part in this faux chase, to join them in their laughter, is without hyperbole, a dream come true.
I don’t close my eyes, as I don’t want Erin to catch me taking in this moment; I’m scared it will ruin it. I drink it in, as best I can, while still participating.
I’m such a lucky man.
Tonight, before our bedtime, Declan wakes up. He’s only slightly fussy at first, but over time ramps up quite a bit. I go in to try to comfort him, to no avail. After a little while, Erin goes in to do the same, and things are no better than when I left him. I wait a bit longer, and go in again.
Normally a very bad daytime sleeper, Declan is usually a champion nighttime sleeper. He goes 11 1/2 hours on most nights, and 12 hours isn’t entirely uncommon. When he does have a bad night, it hits us like a ton of bricks, since it’s so out of the ordinary.
Returning in to Declan this second time, I’m extremely frustrated. I’m trying to get a blog post posted before bed, and this is really cramping my style. I have no answers for him, and he has none for me. This is the pits.
I go in again, spend some time with him, and get him to the point that he’s at least considering sleep again. A small victory. As I sit here now, Declan is still fighting, the end of the battle nowhere in sight. This is shaping up to be a long night.
All of this happened within 3 hours.
Parenting is a rollercoaster. A beautiful, awkward, wonderful, disastrous rollercoaster.
One I hope never ends.
When I was in college, back in the early aughts, my computer was a tower that my father and I had built together. Having found my old website from that time, where I thought it necessary to share this information, I can tell you it was a whopping 1GHz and had 256 MB of RAM. I even had a 250 MB internal ZIP drive and an IBM flat-panel display. This was back when you didn’t see them detached from laptops. I was hot stuff. Well, I thought so anyway.
Before the end of college, I got a ThinkPad T30. I ran both machines in parallel. In the dark ages before Dropbox, this was a total pain. But I made it work, mostly using Windows Briefcases.
Fast forward to a few weeks ago, and I had been rocking a 15" MacBook Pro for work for three years. My own computer was a non-retina, high-res, anti-glare, late 2011 MacBook Pro. This computer hadn’t left my desk for any real stretch of time since I received my work MacBook Pro three years ago. Since my MacBook Pro didn’t have a SSD, I found it intolerable to use.
This year, I decided it was time for something new.
Thinking about my computing needs, I began to wonder: should I really get a laptop again? Or is it time for me to come crawling back to desktops?
I have a brand-new iPad Mini 4 which I love. I have this 2011 MacBook Pro I could always put a cheap SSD in. I have Erin’s MacBook Air I can use in a pinch, as long as I keep liquids away from it. What’s tying me to a laptop other than momentum?
Having heard my friends Marco, Dave, and Jason rave about their 5K iMacs, especially for the iOS developers, I thought it was time to give it a whirl.
I decided to do what I had previously thought unthinkable: I bought a 5K iMac. Well, I bought two. The first arrived effectively DOA (more on that on my podcast), and was returned. The second has been running flawlessly for a week now.
I ordered the mid-range 27" iMac, with upgraded processor (4.0 GHz), upgraded SSD (1 TB), and 32 GB of aftermarket RAM. I ordered mine with the new Magic Keyboard and Magic Mouse 2.
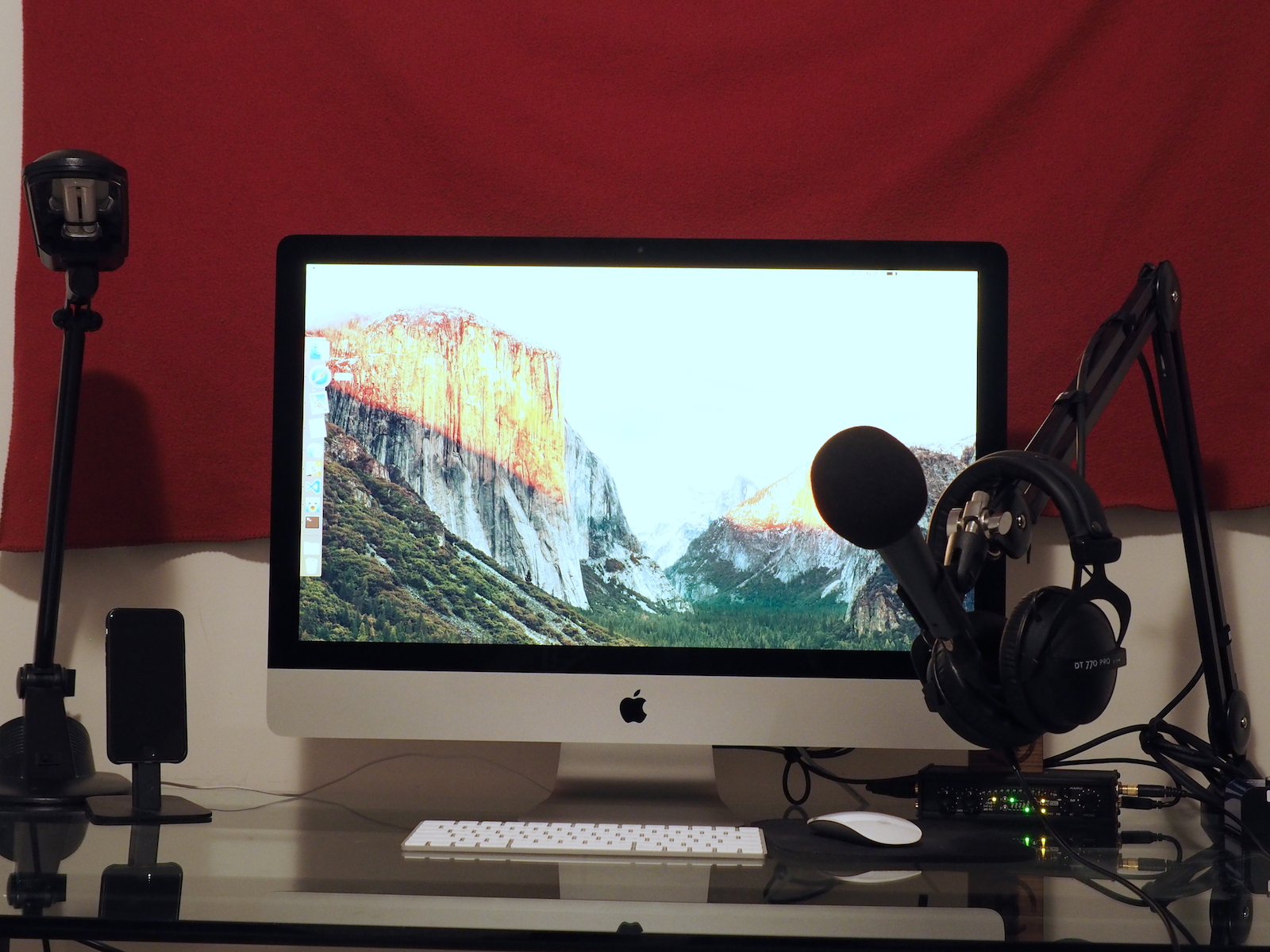
This machine is incredible.
It’s extremely fast, in every measurable way. The SSD is fast. The processor is fast. Everything just seems so fast. Even as compared to my Mid 2015 Retina MacBook Pro.
Most striking is the screen. That seems obvious at first—27 inches is quite a lot of inches. I had played with the iMac at the Apple Store a couple times, and it seemed neat. Once you start actually doing work on one of these machines—start doing the things you normally do—it is quickly obvious how amazing the screen real estate is. I regularly have 20"+ screens connected to my MacBook Pro, and even the combination of the onboard and externals screens seems so cramped now. I’ve been utterly ruined. In a week.
Another interesting artifact of this newfound real estate is that it’s changing the way I interact with OS X. On my laptops, I’m a very heavy Spaces user. I have my virtual desktops set up just right, so that I can keep things separated both mentally and, to some degree, physically as well. What with each of my virtual desktops on the iMac having around three times as many pixels as my MacBook Pro’s, I don’t need virtual desktops near as much.
Don’t look now, but I’m turning into John Siracusa.
The iMac’s accessories are also my first exposure to the new generation of Apple accessories. The Magic Mouse 2 seems about the same to my hand. I know there are differences, but I don’t notice them. The internals I do notice, as I am happy to leave batteries behind for good.
The Magic Keyboard is a marked difference from what I’m used to. I have one of the older, three-battery aluminum Bluetooth keyboards. I’ve always liked Apple keyboards, though curiously, no two ever seem to feel quite alike. Nonetheless, this new, lower travel Magic Keyboard is my favorite of the bunch.
I like it to type on, but I also quite like the lower profile. Since it doesn’t have to make room for two (or three) cylindrical AA batteries, it’s far flatter, which I find more comfortable, if a bit unusual.
I also quite like the newly decreased key travel. I don’t mind how shallow it is at all. Having tried the MacBook One in stores, I found that keyboard mostly agreeable, the spacebar and Return keys excepted. The Magic Keyboard reaches a perfect compromise between the keys I’m used to and the ones in the MacBook One.
There is a catch.
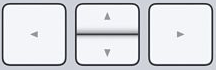
The new arrow keys are driving me batty. Unbeknownst to me, my fingers have always found the arrow keys by looking for the dead space above the half-height left and right arrows. On the Magic Keyboard, the left and right keys are now full-height. My fingers find this terribly unbalanced, as the up and down arrows are still half-height. I’m sure I’ll get used to it over time; a week in, I’m not there yet.
A week in, I haven’t found any reason to regret getting a desktop. There have been times I’ve wanted to sit next to my wife and work on something—like this blog post—but just saving it for when I’m really concentrating seems like the better answer anyway. Then I can give Erin the attention she deserves, as well as what I’m doing on the iMac once I sit down in front of it.
Perhaps Jason put it best:
This is the promise of the 27-inch iMac with Retina 5K Display: It’s one of the fastest Macs ever attached to the best Mac display ever. Yes, it’s an iMac, meaning you can’t attach a newer, faster computer to this thing in two or three years. But I have a feeling that these iMacs will have the processor power, and the staying power, to make the aging process much less painful.