A couple of weeks ago I joined my buddy Jelly (of GIFWrapped fame) on his podcast about mobile app development, Mobile Couch. Since Jelly’s normal co-host—and other friend of mine—Ben was on assignment, I got to fill in.
On this episode, Jelly and I discussed both migrations from Objective-C to Swift, as well as my new forays into functional reactive programming using RxSwift.
It’s really hard to verbally describe the magic that is Rx, but I had a lot of fun recording this episode. Come for the nerd talk; stay for Jelly’s delightful accent.
This week I had my annual appointment at my optometrist. I needed to get fitted for new RGP contacts due to my Keratoconus. During my visit, he placed drops in my eyes to help his examination.
These drops rendered me farsighted for a couple hours. Crisp details were hard to make out up close. I immediately noticed this the moment I tried to use my iPhone. I couldn’t read anything on it.
I didn’t fret. I know that Apple has built a plethora of accessibility tools
into iOS. I began by taking a page out of my parents’ playbook, and increasing
the size of text on the screen. To do so, I went to
Settings
→ General
→ Accessibilty
→ Larger Text
and slid the slider as
far as it would go. Thankfully I knew approximately where all of these items
were, as it was very hard to read them. Cranking the text size way up made
things better:
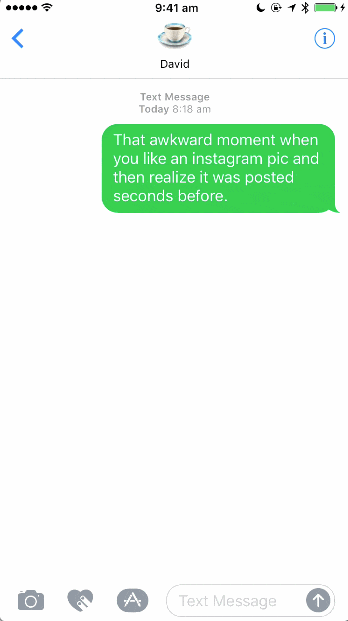
However, this wasn’t really enough. When I recorded the video above, with full
visual acuity, I could read the Larger Accessibility Sizes
switch. For demo
purposes, I turned it on in the video above to show the difference.
At the time, not remembering nor being able to see the larger sizes option, I
wasn’t able to use it. I was able to just barely make out the word Zoom
in the Accessibility screen. Once I looked at the Zoom
details, I was vaguely
able to see something about three fingers
in the text below the switch. I
turned it on, and immediately the display zoomed in.
Intuitively, double-tapping with three fingers zoomed back out. Now that I knew how to turn it on and off, I could zoom in to read the full instructions. By dragging with three fingers, I could pan around while remaining zoomed. This was the piece I needed.
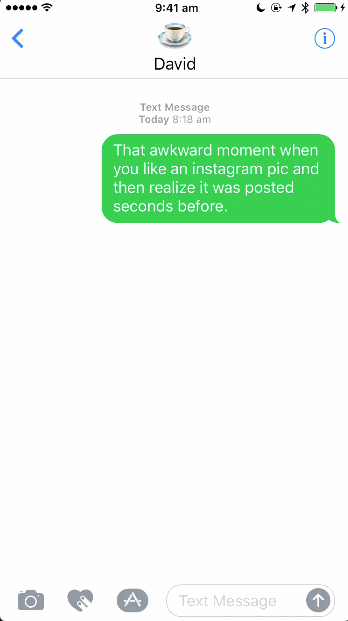
In the span of a couple minutes, without instruction, I went from being unable to see anything on my phone to being able to operate it nearly as efficiently as when I’m able to see just fine. Despite diving into these features quite literally blind with poor vision and no prior experience.
Some have been writing about accessibility—particularly for the visually impaired—for years. Until I found myself in a similar (though temporary) predicament, I didn’t realize nor appreciate how much work Apple puts into making our devices usable by everyone.
Today I joined Katie Floyd, Dan Moren, and Jason Snell on this week’s Clockwise. As usual, the four hosts discussed four topics in thirty minutes.
This week, we discussed new features in macOS Sierra, what we still long for from our phones, what we nevertheless appreciate about our phones, and Google Allo.
Clockwise is so much fun to guest on. If you haven’t listened, you should give it a shot.
The Grand Tour, spiritual successor to the BBC Top Gear that we knew and loved, has set a release date of Friday, 18 November:
The show is filming in different locations across the globe; they film in California the weekend of 24-25 September. I was actually interviewed via phone to potentially win tickets to the show, but more on that later.
The show will be broadcast on Fridays on Amazon Prime. Unlike most other online-only shows, particularly popular Netflix shows like House of Cards and Orange is the New Black, The Grand Tour will not be released in one large chunk. One episode per week, for a few weeks.
We’ve also found out a little bit more about The Grand Tour. According to Jalopnik, there’s a plethora of things that can’t be said or done on the new show:
- No Stig
- No handwritten leaderboard
- No test track
- No saying landscapes are beautiful
- Possibly no use of the word “🐔” by James
On the plus side, according to series producer Andy Wilman in the video Jalopnik discovered:
- The Grand Tour will be filmed in 4K
- There will be twelve episodes “per year”; not sure if that’s per season or per annual year. I’d guess the latter, which means 6 episodes per season.
- The contract is presently 3 years, so that means 36 new shows (!)
About that interview.
On Friday, 2 September, I received the following email:
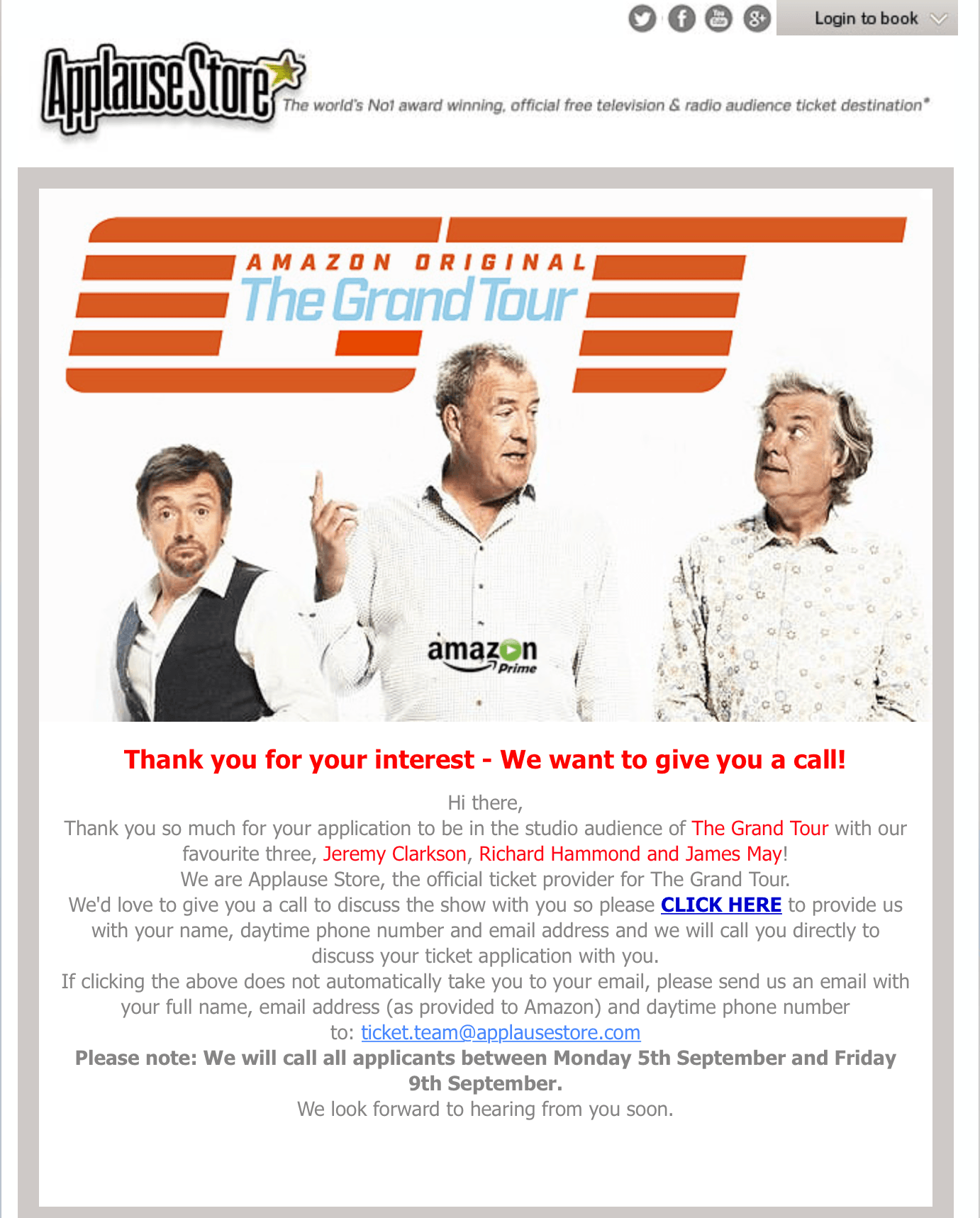
It asked me to provide contact information so that someone from the Applause Store
(which also handled tickets for Top Gear) could
call me ring me up and talk.
On the following Monday, which was Labor Day, I received a call from Unknown
.
That call was from a very lovely British lady; it lasted six minutes.
I was asked a variety of questions. Some made sense, others were odd. The ones I remember:
- Would you consider yourself a big fan of Top Gear?
- What do you like most about it?
- Have you seen the new Top Gear, with the new cast?
- Have you ever been to a car show?
- Have you ever been to a music festival? (ಠ_ಠ)
- I see you’re in Virginia. Could you be in southern California the weekend of 24-25 September, on two weeks notice?
- Who would you bring with you? (I suspect this was to ensure gender parity)
- What’s your favorite car?
I gave the best answers I could. I did make mention of Top Gear Party, but neglected to mention my vanity license plate is inspired by one of the members of the show. I did my best to sell myself, without being too over the top.
I never heard back from the Applause Store, so it sounds like I won’t be making an emergency trip to Southern California.
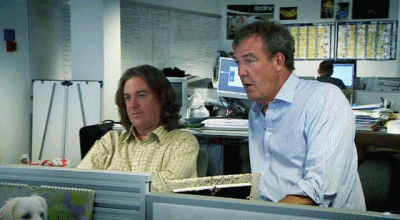
You can’t win 'em all.
Nonetheless, I am super pumped for November.
I just ordered a new iPhone, last night this morning, at 3:11 AM. I got an iPhone 7, [matte] Black, 128 GB. It should ship on launch day.
In placing the order, I learned several things, which I’m putting here mostly to help me remember next year.
The day before
Apple shuts the store down the afternoon/evening of the day before preorders begin, so it’s important to get these steps done ahead of time.
- It’s best to use the
Apple Store
app to place your order, on the device that you will be replacing. It’s a bit cruel to use your current device to order its replacement, but it’s fastest. Using the web is almost always a recipe for misery. - If at all possible, set up Apple Pay on that device.
- Confirm your default shipping and payment information:
Apple Store
app:Account
tab →Primary Payment
andPrimary Shipping
- Apple Pay:
Settings
app →Wallet & Apple Pay
→Shipping Address
- Speaking of shipping address:
- If possible, choose your place of business. The delivery will require a signature, and you don’t want to be trapped at home all day.
- That said, AT&T requires you to ship new devices to the address they have on file. Good luck if you’re on a multi-city family plan.
- Back in the
Apple Store
app, find the device you want, and configure it (go through all the motions like you’re going to buy it right now), and save it as a favorite. - Also make sure you’ve enabled the
Apple Store
app keeping you logged in, as well as your carrier settings. There’s one switch to flip for both:
Account
tab →Account Settings
→Remember Me
The night of
Please note that things won’t be exactly the same for those upgrading via the iPhone Update Program, as of the iPhone 7 you must make an in-store appointment to surrender your 6S, and collect your 7. I’ve also heard second-hand reports that availability was extremely limited for upgraders. Your mileage may vary.
- Wake up a few minutes before preorders go on sale. They are supposed to start at midnight Pacific time, but in reality it’s often 5-10 minutes later. I set my alarm at 2:58 AM for a (roughly) 3 AM sale time.
- Use your cellular connection when you wait for the Apple Store to come back online. I found my otherwise-fantastic FiOS connection didn’t see the store come back up as quickly.
- Though the Apple Store app does (sometimes) refresh itself when you simply leave it and return to it, I’d recommend force-quitting it, reopening it, repeat. That forces the app to refresh and see if the store is up or not.
- When the store comes back up, go to
Account
tab →My Favorites
and proceed from the favorite you saved earlier. It’ll save you time. - When you check out, pay with Apple Pay. It’s faster and increases your chances
of getting your order through quickly.
- Be careful, though, that the correct addresses and e-mails are used.
I found that an old, busted, e-mail address was somehow used for my order,
despite me having confirmed my settings in the
Apple Store
app ahead of time.
- Be careful, though, that the correct addresses and e-mails are used.
I found that an old, busted, e-mail address was somehow used for my order,
despite me having confirmed my settings in the
- For Americans, on the iPhone 7 anyway, if you’d like to get an unlocked, SIM-free
phone, your only choice is T-Mobile. Unlike all the other carriers, they do
not ask for any sort of account confirmation during the order process.
- However, in the case of the iPhone 7 anyway, those phones do not support CDMA carriers Sprint and Verizon.
The wait
I cannot recommend Deliveries enough to track your order. They have apps for all the Apple platforms, and they’re all great. Deliveries supports tracking by Apple order number.
Additionally, often times a day or two before your target delivery date, you can actually get your tracking information before Apple sends it to you. It changes often whether Apple uses FedEx or UPS to ship the phones, but either way, go to the tracking page for either carrier.
Tracking by reference
Once you’re on the tracking page, try to track a package by reference. Try using either your billing phone number or your iPhone’s phone number as that reference number. (I’ve seen both used in the past). Sometimes, this will give you your iPhone tracking information. Sometimes, it doesn’t. Can’t hurt to try.
In the case of UPS, which Apple has used for me in recent years, it’s worth
noting that you must be on the desktop site to track by reference.
Additionally, you may find that you can also use your Apple order number,
with the last two characters truncated, as a reference number. For example,
for order W123456789
, try to track by reference W1234567
.
UPS My Choice
For the iPhone 7, I signed into my free UPS My Choice account in order to see if the tracking number was visible. When I went to my Delivery Planner calendar, I noticed on Wednesday (the first time I looked) that my phone had a tracking number and was scheduled for Friday.
Arrival day
It’s worth noting that in years past, if you’d like, Apple has allowed you to print and pre-sign a form and tape it to your door. You can print the signature form PDF from your order status page. The delivery person is supposed to take that as proof of signature, and leave you your phone. Depending on the area in which you live, this may be risky, as the iPhones ship in boxes that look like they have iPhones in them.
- Upgrade your existing phone to the latest version of iOS—in this case, iOS 10—before you do anything. Since the iPhone 7 will presumably ship with 10, it’ll make the whole backup dance much easier.
- Unpair your Apple Watch, if you have one, from your iPhone
Additionally, if you have an Apple Watch with LTE, unless you’re switching carriers, choose to keep your service. More in this kbase article. - Perform a backup of your iPhone, preferably to iTunes, preferably with
Encrypt iPhone backup
checked, so that your passwords will restore automatically on the new phone - Go through the onboarding on the new iPhone
- Restore the backup you created in step #2
- Re-pair your Apple Watch with your new iPhone.
Here’s hoping your purchase experience—and mine!—is as smooth as possible in 2017.
UPDATED 10 September 2016 2:00 PM: Added some thoughts about the iPhone Upgrade Program, updating your existing phone, and pre-signing for delivery.
UPDATED 13 September 2016 07:45 AM: Added information about using UPS My Choice to get your tracking number.
UPDATED 1 November 2017 11:00 PM: Added information about Apple Watch with LTE, and tracking by reference.
Today, Apple is going to be unveiling the next version of the iPhone. I’ll be joining my buddies Jason Snell and Dan Moren of Six Colors, as well as Stephen Hackett, and Myke Hurley, on a live chat hosted by Talk Show.
I’ve embedded the chat below, but you can also find it here, or in the native Talk Show app. We’re doing a Q&A before the event, and then join us while we discuss the keynote, 1 PM today!
Today I joined Dan Moren, Jason Snell, and Brianna Wu on this week’s Clockwise. As with all episodes of Clockwise, it’s four topics from four hosts in thirty minutes.
This week, we discussed Amazon’s $5/mo music service for Alexa, Bloomberg’s report on Apple Watch 2, old software we just can’t get rid of, and our policies with beta OSes on our devices.
Guesting on Clockwise is one of my favorite things to do, as I always have a blast. Making a point despite the constant pressure to be very succinct is always a fun challenge. In fact, Brianna described it well in a series of tweets today.
At work last week, I was debugging some odd behavior in one of our apps. A user,
on a detail screen, would tap a Save
button, and nothing would happen. Not a
crash, not an error message, no log entries. Just, nothing.
I started digging into the code, and quickly found we were trying to add this new item represented by the detail screen onto a custom collection. Something along these lines (the names have been changed to protect the guilty):
[self.customCollection addItem:self.collectionItem];
As it turns out, a couple of classes above this one, the instance of customCollection
wasn’t being properly initialized; it was simply nil
.
That means in the code above, we were passing a message to nil
. In Objective-C,
that means the message is dropped on the floor, and ignored.
In many other languages, such as C♯, calling a method on a null
variable
would cause a crash. Messaging to nil
being acceptable is considered a
feature in Objective-C, and not a bug. Crashes are never a good thing,
after all.
In Swift, by comparison, we can only have nil
values on Optional
s.
We do have the handy optional chaining syntax, however, that would change
the code above to:
self.customCollection?.add(item: self.collectionItem)
As a Swift developer, seeing that ?
would immediately cue to me that it’s
possible for customCollection
to be nil
. Something that is worth investigating.
That being said, any Objective-C developer worth their salt will think the same
of any class instance. To a Swift developer, that sounds exhausting, but it’s
still a reasonable point. Further, many Swift developers only use optional
chaining in cases they’re pretty damn sure things will either be non-nil
, or
it’ll work itself out if things are nil
. The ?
may get overlooked.
In our case, where nil
really is bad news, a good Swift developer would improve
upon the above:
guard let collection = self.customCollection else {
// Take evasive action, or just punt:
fatalError("Custom Collection cannot be null!")
}
// If we get here, we are *guaranteed*
// that collection is non-nil.
collection.add(self.collectionItem)
Here, it’s made explicitly obvious what’s happening: the guard let
indicates
this thing had better be true, or else bad things have happened.
“But wait!” shouts the Objective-C developer. “We have NSAssert
!”
Okay, sure. But NSAssert
isn’t always enabled; in fact, in general, it’s
disabled by most in release builds.
The point isn’t that Swift’s Optional
s or guard let
have no equivalents
in Objective-C. The point is that thinking deliberately about these
things—and proactively protecting yourself from error conditions—is
a fundamental part of how you write Swift.
Some people may find being that concerned with what is Optional
and what isn’t
to be exhausting. I find it to be lovely. I find myself being far more
deliberate with the Swift code that I write. In many ways, it’s reminiscent of
the C♯ I wrote in the past, but with better tools such as guard let
and
optional chaining (which was just added in C♯ 6.0, actually).
I like Swift, and I like being that careful with my code. Swift instills a care in me that I like to think is innate, but is now compulsory.
It all started with an uncomfortable discovery:
Are PPTP VPNs not available in iOS 10‽
— Casey Liss (@caseyliss) July 17, 2016
(I’m aware of the security concerns, but, bummer.) pic.twitter.com/nTWeTKg1LX
I was previously using a PPTP VPN to allow myself to tunnel into my home network from work or when I’m out. I don’t use the VPN terribly often, but I consider it critical enough that I couldn’t stand to be without it.
Since PPTP is, by anyone’s measure, woefully insecure, Apple has removed it from iOS 10 and macOS Sierra. Thus, I needed to make a change.
I have a Synology DS1813+ (now replaced by the 1815+), which is an absolutely brilliant home server, in addition to being a dumping ground for all my e-hoarding. Among the Synology’s many features is acting as a VPN server. In fact, it was the machine hosting the PPTP VPN server.
The Synology offers two alternatives to PPTP: L2TP/IPSec and OpenVPN. For the former, L2TP is simply a tunneling protocol; IPSec provides the security. For the latter, OpenVPN covers everything, but does not have native, out of the box support on Apple operating systems. To me, that meant it was a non-starter.
On the Synology side, thanks to the work I had already done, I was able to configure L2TP by checking a checkbox and setting a shared secret. To connect to the VPN, I need not only my user’s account password, but also a shared secret that is, well, shared amongst all users.
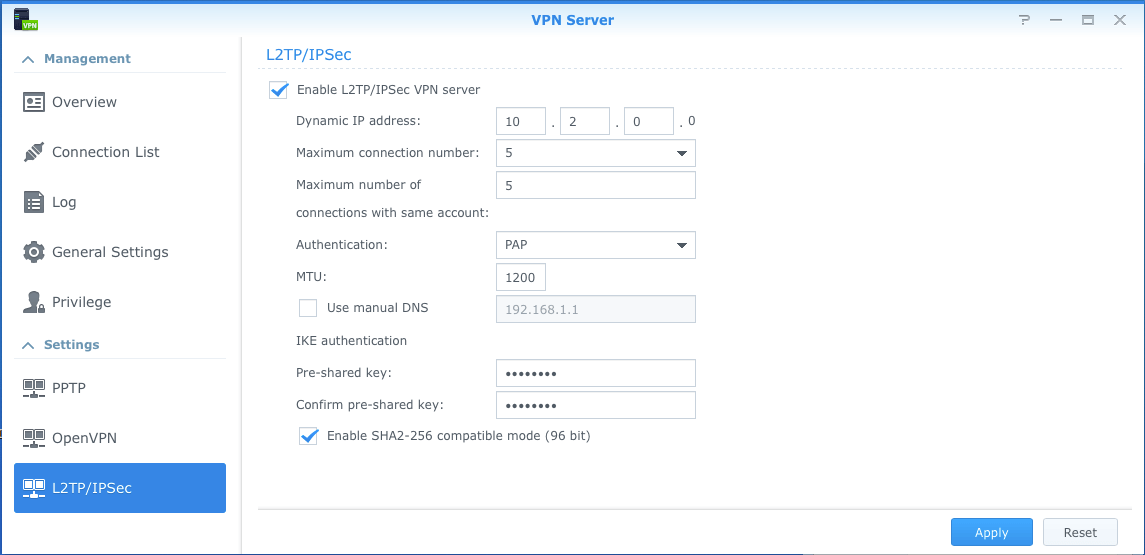
That part was easy.
However, I kept having problems connecting from my work MacBook Pro. For the life of me, I couldn’t figure out why, but I felt like it was a firewall issue. I eventually confirmed it to be so by just doing the obvious and attempting to connect to the VPN from within my network.
As noted on Synology’s website, L2TP requires the following ports to be open:
- UDP 1701
- UDP 500
- UDP 4500
However, what isn’t made clear is that some protocols need to be forwarded as well. I discovered this thanks to this very difficult to parse post on the Verizon forums. It seems to me the following protocols also need to be forwarded:
I am a Verizon FiOS user, and because I didn’t know better at the time it was installed in 2008, my internet connection is through a coax line. Though it’s possible to insist to your Verizon installer that they use ethernet to deliver your internet connection inside the house, mine was done over coax, and I don’t want to be bothered changing it.
The only problem that comes of this—which isn’t much of a problem at all—is that I have to use a router that has a coax connection on it. Which means I’m still using the same router that I received in 2008. That said, it operates flawlessly, and has only ever caused me trouble this time, when I attempted to configure the port forwarding rules for L2TP.
The appropriate way to configure the Verizon FiOS ActionTek router for L2TP/IPSec is as follows.
Begin by setting up a new port forwarding rule. Out of the box, the ActionTek comes set up with rules for many (then-) modern services. Setting up a new rule allows for the several ports/protocols to be grouped together as one. However, critically, a port forwarding rule is the only way to forward the GRE protocol.
To set up a new port forwarding rule, begin by logging into your router,
likely by pointing your web browser to http://192.168.1.1/
. Using the
“tabs” at the top, choose Advanced
. In the bottom, you will see
Port Forwarding Rules
; choose that:
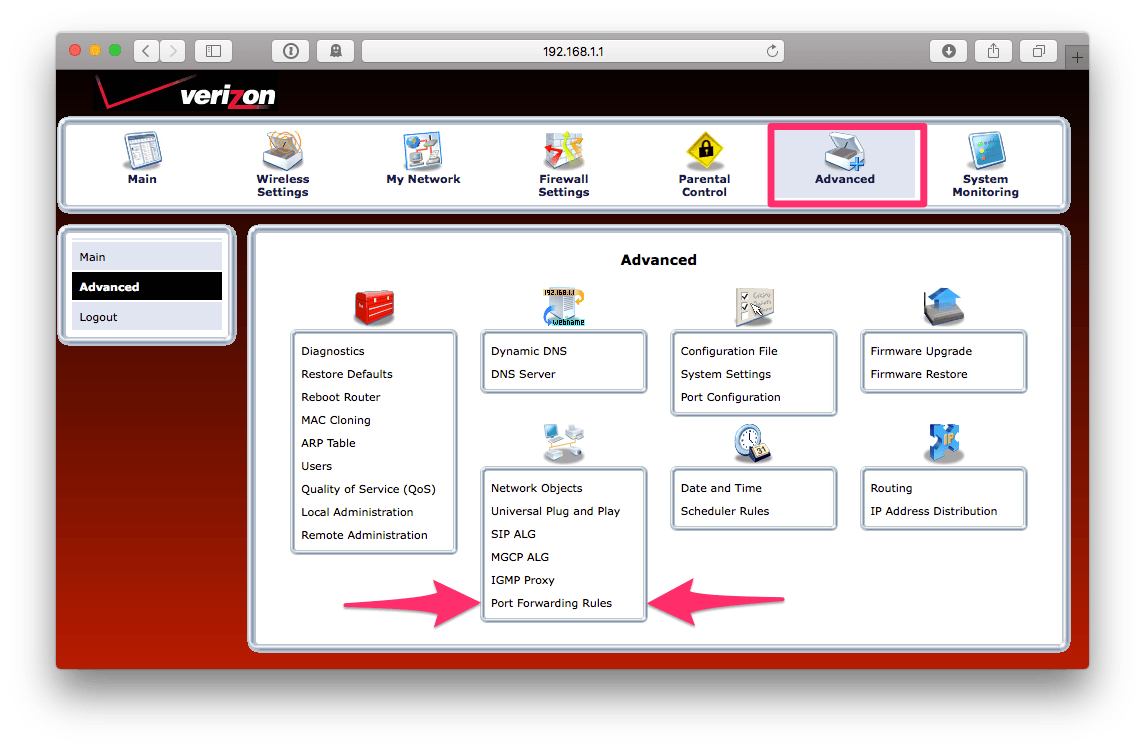
Here, we will create a new rule. You can do so by scrolling all the way to the
bottom, and finding the little Add
link:

Now we can start adding ports and services, much like it works when setting up a normal port forwarding record. Notice that here, we can choose protocols as well as ports (by way of their TCP/UDP protocols):
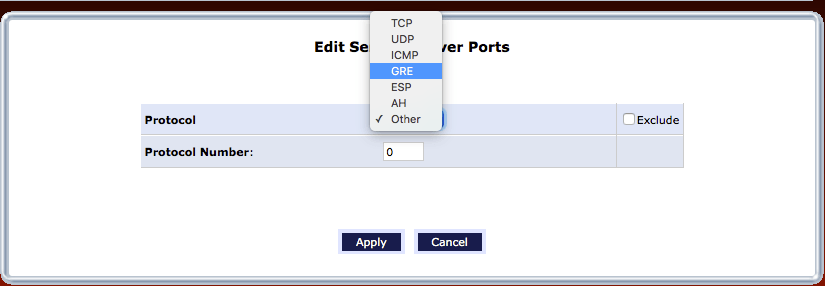
When everything is configured, it should look something like this:
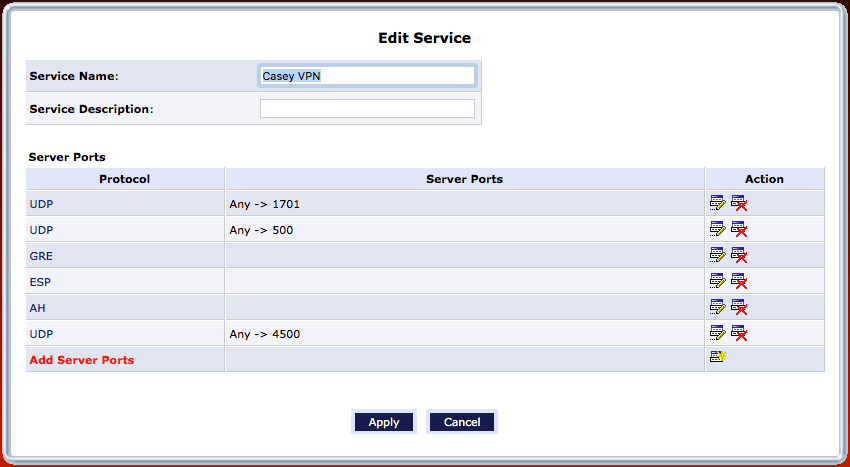
Now this new port forwarding rule can be leveraged, and it can be pointed at
the Synology. In the “tab bar”, choose Firewall Settings
and then, on the
left, Port Forwarding
. In the leftmost drop down at the top, select the
IP for the L2TP host. In the Application to forward
drop down, the new
VPN setting should be an option; in my case, it’s Casey VPN
:
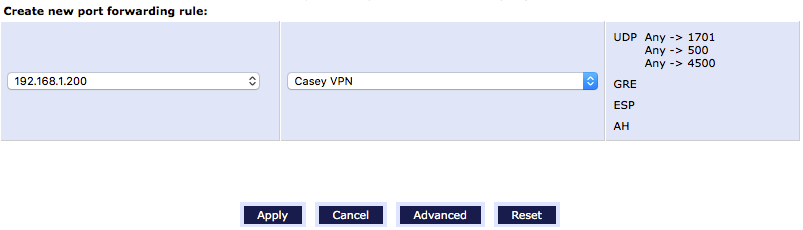
Click Apply
, and everything should be all set.
On the macOS and iOS sides, the new VPN connection can be set up as a standard L2TP VPN. Just be sure to enter the shared key and password exactly right.
Now I’m ready for the new operating systems this fall, and as an added bonus, I’m more secure today.
Nothing is worse than having an itch you can only scratch by playing a particular song from a particular concert film, and then having to seek through the entire 1-2 hour file to get to it.
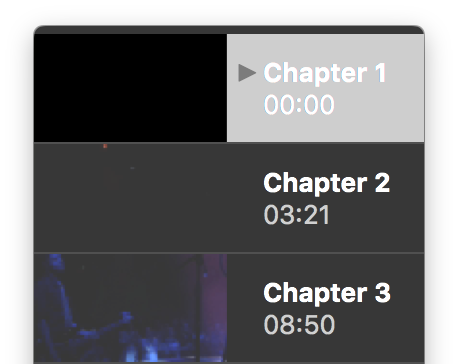
My general workflow for digitizing my DVDs and BluRays is as follows:
- Rip the disc with MakeMKV
- Transcode the resultant MKV to MP4 using Don Melton’s Scripts
- Name appropriately
Between MakeMKV and Don’s scripts, any chapter markers in the original disc are
preserved in the resultant MP4. However, they are named as one would expect, and
as shown above: Chapter 1
, Chapter 2
, etc.
Enter Subler.
Subler has many uses, including remuxing files between formats without a full transcode, in some cases. However, the feature of Subler that’s useful in this context is that it allows me to go through the file that Don’s scripts generated, and rename the chapters.
After a little work on my part figuring out which chapter is which song, Subler lets me transform the above to this:
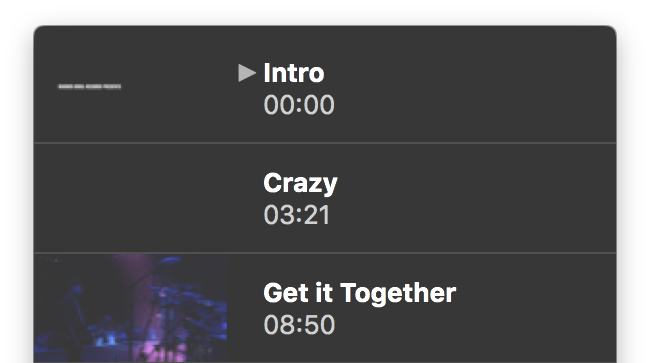
In the rare case the source material didn’t have chapters, I can even take the liberty of adding them. In the Subler window:
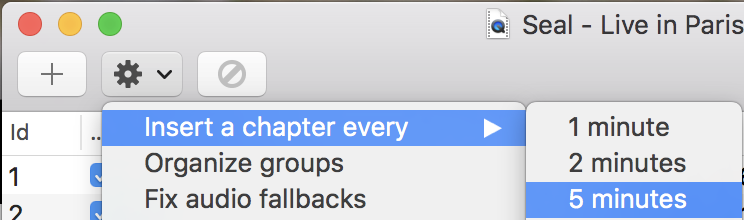
That will give you a decent place to start from. Unfortunately, you’ll have to scrub through to find the exact times of each song, but you’ll only have to do it once.
When you’re all done, you should see something like this:
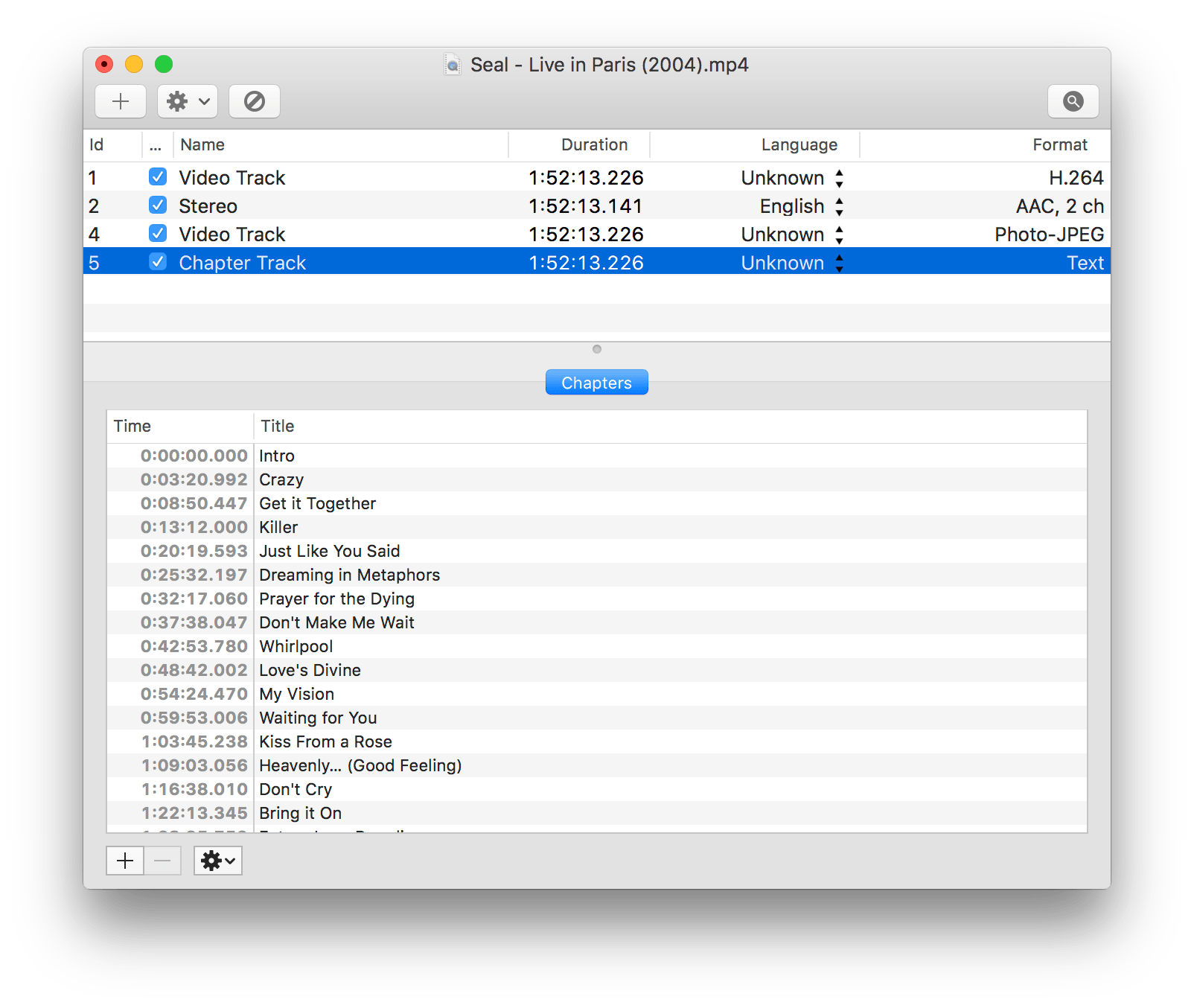
Tell Subler to save the file, and it will overwrite the existing one in place, in just a few moments. No transcoding required, unless your source is something that doesn’t support chapters and/or chapter names.
In my case, once these files are post-processed, I drop them in a place that Plex can see them. When I play them back on my Apple TV, I swipe down on the remote to see the list of chapters. On the Plex app, there is an icon on screen to jump between chapters.
A little time up front can prevent a total buzzkill later.